原圖:
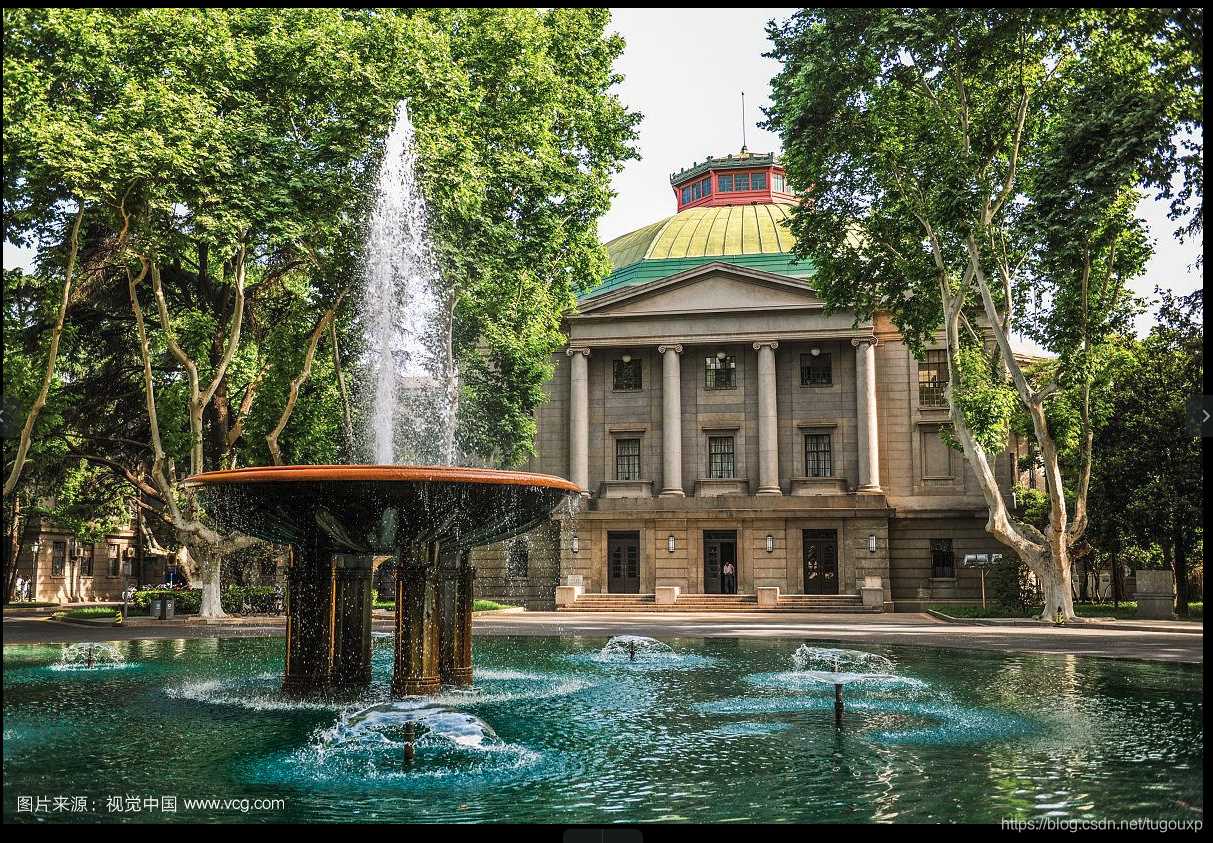
圖像信息,可以看到圖像是一個816*2100像素的圖片:
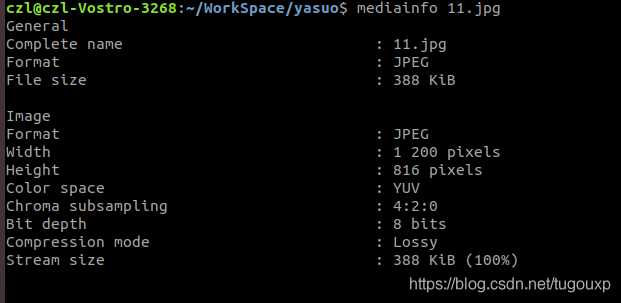
python代碼:
import cv2
import numpy as np
import matplotlib.pyplot as plt
img = cv2.imread('11.jpg', 0)
img1 = img.astype('float')
img_dct = cv2.dct(img1)
img_dct_log = np.log(abs(img_dct))
img_recor = cv2.idct(img_dct)
recor_temp = img_dct[0:100,0:100]
recor_temp2 = np.zeros(img.shape)
recor_temp2[0:100,0:100] = recor_temp
print recor_temp.shape
print recor_temp2.shape
img_recor1 = cv2.idct(recor_temp2)
plt.subplot(221)
plt.imshow(img)
plt.title('original')
plt.subplot(222)
plt.imshow(img_dct_log)
plt.title('dct transformed')
plt.subplot(223)
plt.imshow(img_recor)
plt.title('idct transformed')
plt.subplot(224)
plt.imshow(img_recor1)
plt.title('idct transformed2')
plt.show()
僅僅提取一個100*100的DCT系數(shù)后的效果:

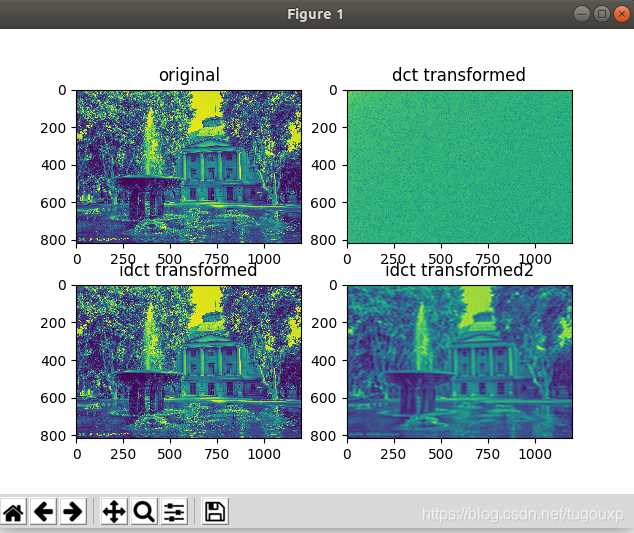
當用800*1000的DCT系數(shù):

可以看到圖像細節(jié)更豐富了一些:
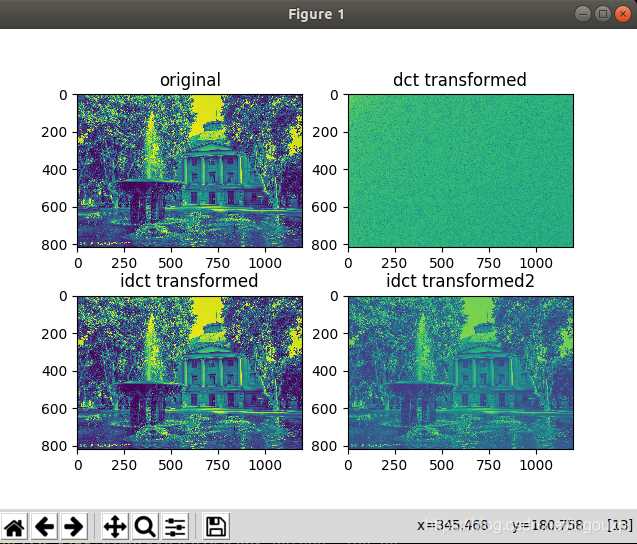
import cv2
import numpy as np
import matplotlib.pyplot as plt
img = cv2.imread('11.jpg', 0)
img1 = img.astype('float')
img_dct = cv2.dct(img1)
img_dct_log = np.log(abs(img_dct))
img_recor = cv2.idct(img_dct)
recor_temp = img_dct[0:800,0:1000]
recor_temp2 = np.zeros(img.shape)
recor_temp2[0:800,0:1000] = recor_temp
print recor_temp.shape
print recor_temp2.shape
img_recor1 = cv2.idct(recor_temp2)
plt.subplot(221)
plt.imshow(img)
plt.title('original')
plt.subplot(222)
plt.imshow(img_dct_log)
plt.title('dct transformed')
plt.subplot(223)
plt.imshow(img_recor)
plt.title('idct transformed')
plt.subplot(224)
plt.imshow(img_recor1)
plt.title('idct transformed2')
plt.show()
當用816*1200的DCT系數(shù):

可以看出圖像恢復到原來的質量了.
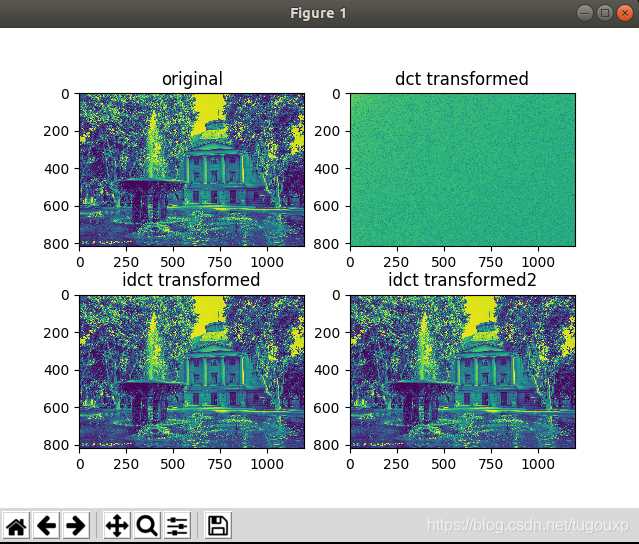
分析代碼:
img_dct保存的是dct變換后的矩陣,img_dct_log是矩陣中的元素首先取絕對值,再求對數(shù)的矩陣.
img_dct_log = np.log(abs(img_dct))
那么對數(shù)的底是多少呢?
打印出來img_dct_log和abs(img_dct)看一下:
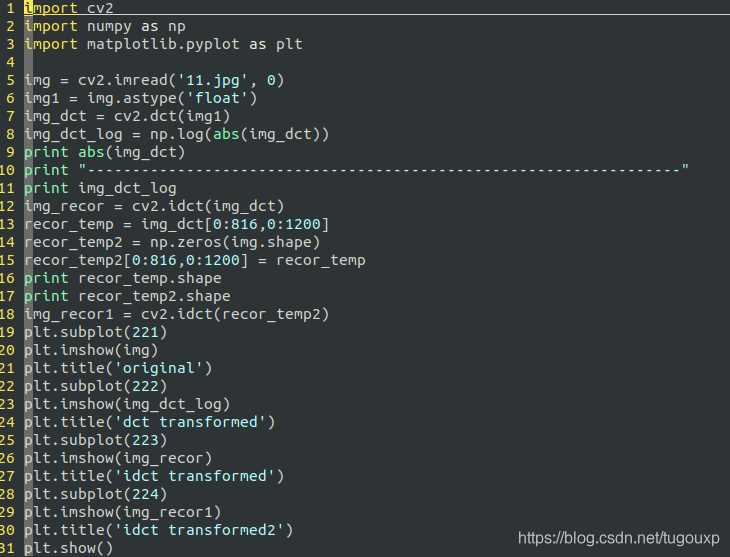
打印結果:
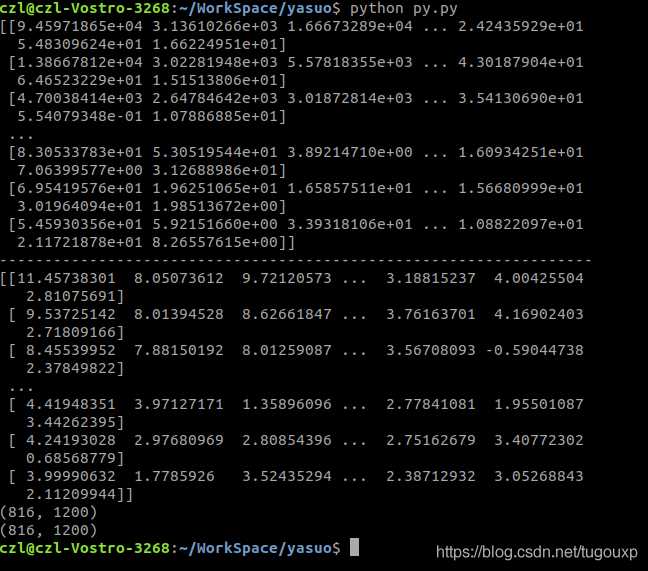
其中9.45971865e+04=9.45971865 x 10^4 =94597.1865表示的是科學計數(shù)法.
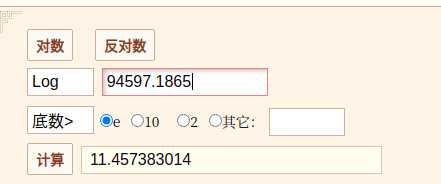
我們看到只有在底數(shù)取e的時候,對應的對數(shù)才符合題目輸出要求,所以,python numpy.log函數(shù)取的是以自然常數(shù)e為地的對數(shù).
到此這篇關于OpenCV實現(xiàn)圖片編解碼實踐的文章就介紹到這了,更多相關OpenCV 圖片編解碼內(nèi)容請搜索腳本之家以前的文章或繼續(xù)瀏覽下面的相關文章希望大家以后多多支持腳本之家!
您可能感興趣的文章:- python opencv通過按鍵采集圖片源碼
- Opencv判斷顏色相似的圖片示例代碼
- C++ opencv ffmpeg圖片序列化實現(xiàn)代碼解析
- 用opencv給圖片換背景色的示例代碼
- python opencv把一張圖片嵌入(疊加)到另一張圖片上的實現(xiàn)代碼
- python opencv實現(xiàn)gif圖片分解的示例代碼
- python opencv圖片編碼為h264文件的實例
- 10個步驟Opencv輕松檢測出圖片中條形碼