問(wèn)題
假設(shè)你在存檔中有成千上萬(wàn)的文檔,其中許多是彼此重復(fù)的,即使文檔的內(nèi)容相同,標(biāo)題不同。 現(xiàn)在想象一下,現(xiàn)在老板要求你通過(guò)刪除不必要的重復(fù)文檔來(lái)釋放一些空間。
問(wèn)題是:如何過(guò)濾標(biāo)題足夠相似的文本,以使內(nèi)容可能相同? 接下來(lái),如何實(shí)現(xiàn)此目標(biāo),以便在完成操作時(shí)不會(huì)刪除過(guò)多的文檔,而保留一組唯一的文檔? 讓我們用一些代碼使它更清楚:
titles = [
"End of Year Review 2020",
"2020 End of Year",
"January Sales Projections",
"Accounts 2017-2018",
"Jan Sales Predictions"
]
# Desired output
filtered_titles = [
"End of Year Review 2020",
"January Sales Projections",
"Accounts 2017-2018",
]
根據(jù)以上的問(wèn)題,本文適合那些希望快速而實(shí)用地概述如何解決這樣的問(wèn)題并廣泛了解他們同時(shí)在做什么的人!
接下來(lái),我將介紹我為解決這個(gè)問(wèn)題所采取的不同步驟。下面是控制流的概要:
預(yù)處理所有標(biāo)題文本
生成所有標(biāo)題成對(duì)
測(cè)試所有對(duì)的相似性
如果一對(duì)文本未能通過(guò)相似性測(cè)試,則刪除其中一個(gè)文本并創(chuàng)建一個(gè)新的文本列表
繼續(xù)測(cè)試這個(gè)新的相似的文本列表,直到?jīng)]有類似的文本留下
用Python表示,這可以很好地映射到遞歸函數(shù)上!
代碼
下面是Python中實(shí)現(xiàn)此功能的兩個(gè)函數(shù)。
import spacy
from itertools import combinations
# Set globals
nlp = spacy.load("en_core_web_md")
def pre_process(titles):
"""
Pre-processes titles by removing stopwords and lemmatizing text.
:param titles: list of strings, contains target titles,.
:return: preprocessed_title_docs, list containing pre-processed titles.
"""
# Preprocess all the titles
title_docs = [nlp(x) for x in titles]
preprocessed_title_docs = []
lemmatized_tokens = []
for title_doc in title_docs:
for token in title_doc:
if not token.is_stop:
lemmatized_tokens.append(token.lemma_)
preprocessed_title_docs.append(" ".join(lemmatized_tokens))
del lemmatized_tokens[
:
] # empty the lemmatized tokens list as the code moves onto a new title
return preprocessed_title_docs
def similarity_filter(titles):
"""
Recursively check if titles pass a similarity filter.
:param titles: list of strings, contains titles.
If the function finds titles that fail the similarity test, the above param will be the function output.
:return: this method upon itself unless there are no similar titles; in that case the feed that was passed
in is returned.
"""
# Preprocess titles
preprocessed_title_docs = pre_process(titles)
# Remove similar titles
all_summary_pairs = list(combinations(preprocessed_title_docs, 2))
similar_titles = []
for pair in all_summary_pairs:
title1 = nlp(pair[0])
title2 = nlp(pair[1])
similarity = title1.similarity(title2)
if similarity > 0.8:
similar_titles.append(pair)
titles_to_remove = []
for a_title in similar_titles:
# Get the index of the first title in the pair
index_for_removal = preprocessed_title_docs.index(a_title[0])
titles_to_remove.append(index_for_removal)
# Get indices of similar titles and remove them
similar_title_counts = set(titles_to_remove)
similar_titles = [
x[1] for x in enumerate(titles) if x[0] in similar_title_counts
]
# Exit the recursion if there are no longer any similar titles
if len(similar_title_counts) == 0:
return titles
# Continue the recursion if there are still titles to remove
else:
# Remove similar titles from the next input
for title in similar_titles:
idx = titles.index(title)
titles.pop(idx)
return similarity_filter(titles)
if __name__ == "__main__":
your_title_list = ['title1', 'title2']
similarty_filter(your_title_list)
第一個(gè)是預(yù)處理標(biāo)題文本的簡(jiǎn)單函數(shù);它刪除像' the ', ' a ', ' and '這樣的停止詞,并只返回標(biāo)題中單詞的引理。
如果你在這個(gè)函數(shù)中輸入“End of Year Review 2020”,你會(huì)得到“end year review 2020”作為輸出;如果你輸入“January Sales Projections”,你會(huì)得到“january sale projection”。
它主要使用了python中非常容易使用的spacy庫(kù).
第二個(gè)函數(shù)(第30行)為所有標(biāo)題創(chuàng)建配對(duì),然后確定它們是否通過(guò)了余弦相似度測(cè)試。如果它沒(méi)有找到任何相似的標(biāo)題,那么它將輸出一個(gè)不相似標(biāo)題的列表。但如果它確實(shí)找到了相似的標(biāo)題,在刪除沒(méi)有通過(guò)相似度測(cè)試的配對(duì)后,它會(huì)將這些過(guò)濾后的標(biāo)題再次發(fā)送給它自己,并檢查是否還有相似的標(biāo)題。
這就是為什么它是遞歸的!簡(jiǎn)單明了,這意味著函數(shù)將繼續(xù)檢查輸出,以真正確保在返回“最終”輸出之前沒(méi)有類似的標(biāo)題。
什么是余弦相似度?
但簡(jiǎn)而言之,這就是spacy在幕后做的事情……
首先,還記得那些預(yù)處理過(guò)的工作嗎?首先,spacy把我們輸入的單詞變成了一個(gè)數(shù)字矩陣。
一旦它完成了,你就可以把這些數(shù)字變成向量,也就是說(shuō)你可以把它們畫在圖上。
一旦你這樣做了,計(jì)算兩條直線夾角的余弦就能讓你知道它們是否指向相同的方向。
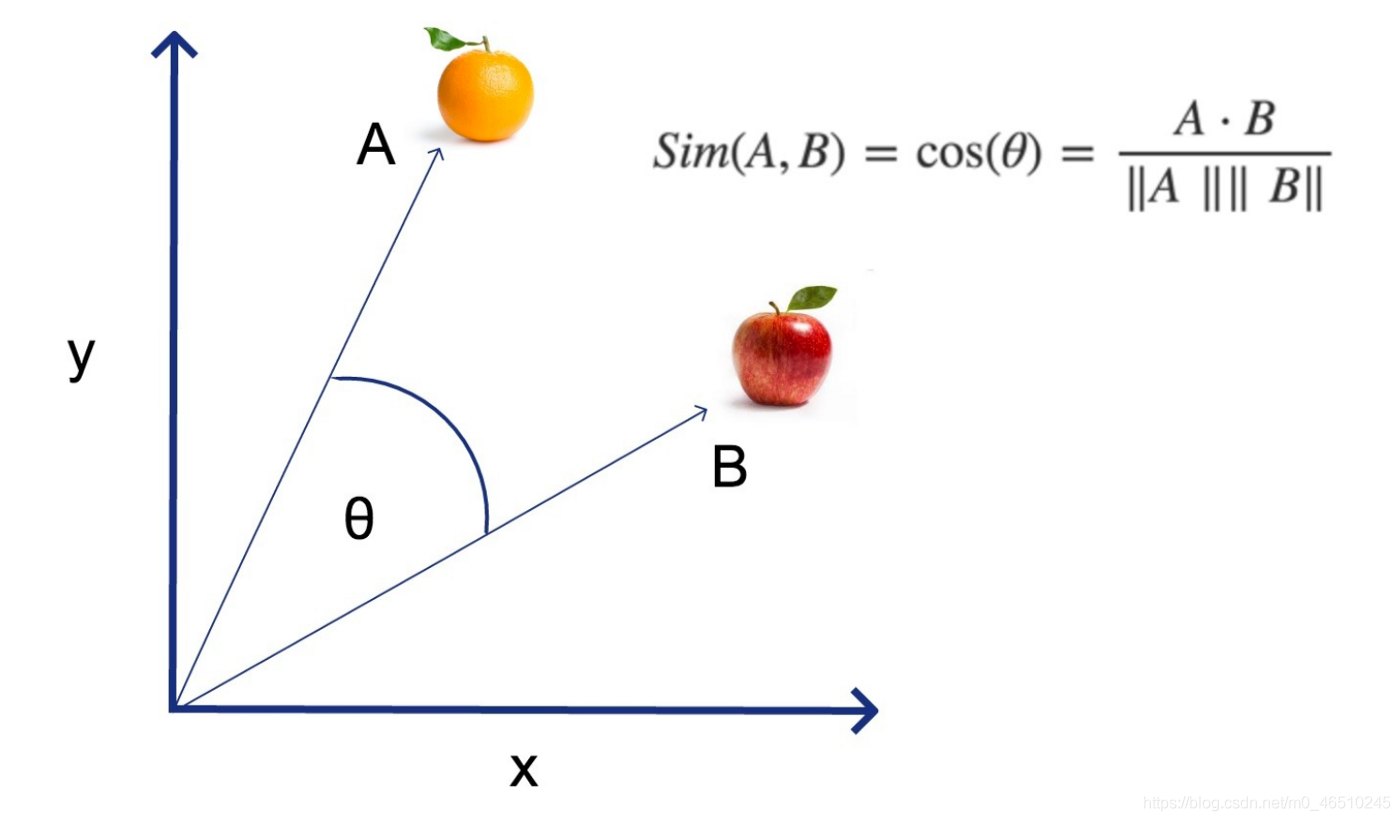
所以,在上圖中,想象一下,A線代表“閃亮的橙色水果”,B線代表“閃亮的紅蘋果是一種水果”。
在這種情況下,行A和行B都對(duì)應(yīng)于空格為這兩個(gè)句子創(chuàng)建的數(shù)字矩陣。這兩條線之間的角度——在上面的圖表中由希臘字母theta表示——是非常有用的!你可以計(jì)算余弦來(lái)判斷這兩條線是否指向同一個(gè)方向。
這聽(tīng)起來(lái)似乎是顯而易見(jiàn)的,難以計(jì)算,但關(guān)鍵是,這種方法為我們提供了一種自動(dòng)化整個(gè)過(guò)程的方法。
總結(jié)
回顧一下,我已經(jīng)解釋了遞歸python函數(shù)如何使用余弦相似性和spacy自然語(yǔ)言處理庫(kù)來(lái)接受相似文本的輸入,然后返回彼此不太相似的文本。
可能有很多這樣的用例……類似于我在本文開(kāi)頭提到的歸檔用例,你可以使用這種方法在數(shù)據(jù)集中過(guò)濾具有惟一歌詞的歌曲,甚至過(guò)濾具有惟一內(nèi)容類型的社交媒體帖子。
到此這篇關(guān)于利用Python過(guò)濾相似文本的簡(jiǎn)單方法的文章就介紹到這了,更多相關(guān)Python過(guò)濾相似文本內(nèi)容請(qǐng)搜索腳本之家以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持腳本之家!
您可能感興趣的文章:- python使用jieba實(shí)現(xiàn)中文分詞去停用詞方法示例
- Python實(shí)現(xiàn)敏感詞過(guò)濾的4種方法
- Python過(guò)濾序列元素的方法
- python numpy實(shí)現(xiàn)多次循環(huán)讀取文件 等間隔過(guò)濾數(shù)據(jù)示例
- python正則過(guò)濾字母、中文、數(shù)字及特殊字符方法詳解
- python基礎(chǔ)之停用詞過(guò)濾詳解