很多企業(yè)做項(xiàng)目使用前后端分離,后端提供接口地址,前端使用接口地址拿數(shù)據(jù),并渲染頁面。那么,前端用戶登錄如何使用接口進(jìn)行認(rèn)證?網(wǎng)上各種教程寫的不堪入目,完全看不懂,所以我根據(jù)自己的理解,寫下此篇文章,希望能幫助到大家。
后端(Laravel5.6框架)
1、使用 composer
安裝 Passport
,打開終端,執(zhí)行命令:
composer require laravel/passport
#安裝完成后,在composer.json文件中會(huì)看到文件版本信息
2、接下來,將 Passport
的服務(wù)提供者注冊到配置文件 config/app.php
的 providers
數(shù)組中
Laravel\Passport\PassportServiceProvider::class,
3、執(zhí)行數(shù)據(jù)庫遷移
php artisan migrate #數(shù)據(jù)庫中會(huì)生成接口認(rèn)證所需的5張表
4、創(chuàng)建密碼授權(quán)客戶端
php artisan passport:client --password
#創(chuàng)建了client_id和client_secret,前端登錄驗(yàn)證的時(shí)候必須把這兩個(gè)玩意兒帶著
5、獲取keys
php artisan passport:keys
6、配置路由
打開服務(wù)提供者 AuthServiceProvider
, 在 boot
方法中加入如下代碼:
use Laravel\Passport\Passport;
public function boot() {
$this->registerPolicies();
Passport::routes(); //接口認(rèn)證的路由
}
然后將配置文件 config/auth.php
中授權(quán)看守器 guards
的 api
的 driver
選項(xiàng)改為 passport
我這里的 customer
表是前端用戶表,但是 laravel
默認(rèn)的是 user
表,所以這里需要做如下配置:
'guards' => [
'web' => [
'driver' => 'session',
'provider' => 'users',
],
'api' => [
'driver' => 'passport',
'provider' => 'customers',
],
],
'providers' => [
'users' => [
'driver' => 'eloquent',
'model' => App\User::class,
],
'customers' => [
'driver' => 'eloquent',
'model' => App\Models\Shop\Customer::class,
],
],
7、注冊中間件,在 app/Http/Kernel.php
文件中的 $routeMiddleware
數(shù)組中添加如下中間件
protected $routeMiddleware = [
'client.credentials'=>\Laravel\Passport\Http\Middleware\CheckClientCredentials::class,
];
然后在需要認(rèn)證接口路由文件 routes/api.php
前面加上這個(gè)中間件。
Route::group(['prefix' => 'cart', 'middleware' => ['client.credentials']], function () {
...
});
8、前端用戶表 customer
模型里面做如下配置:
use Illuminate\Foundation\Auth\User as Authenticatable;
use Laravel\Passport\HasApiTokens;
class Customer extends Authenticatable
{
use HasApiTokens;
....
}
至此,后端的所有配置已完成。
接下來,打開接口測試工具(postman),輸入接口地址: wechat.test/oauth/token
,請求類型 POST
,填上如下參數(shù),點(diǎn)擊 send
你會(huì)看到后臺(tái)返回了前端所需的 access_token
:
前端(vue.js)
首先去加載用戶登錄組件,即用戶登錄頁面。
1. 配置路由,在 index.js
文件中寫入如下代碼
import Login from '@/components/customer/Login'
export default new Router({
routes: [
....
{
path: '/customer/login',
name: 'Login',
component: Login
},
]
})
2、加載組件,在 customer
文件夾的 Login.vue
文件中寫入如下代碼:
template>
div>
input type="email" v-model="customer.email" placeholder="請輸入郵箱">
input type="password" v-model="customer.password" placeholder="請輸入密碼">
button @click.prevent="submit">登 錄/button>
/div>
/template>
script>
export default {
data() {
return {
customer: {
email: '',
password: ''
}
}
},
methods: {
submit() {
//將數(shù)據(jù)配置好
const data = {
grant_type: 'password', //oauth的模式
client_id: 1, //上面所說的client_id
client_secret: 'CO331cA1mqiKgGvvgiDzPxh4CUu19vSEiqxM7LHD',//同上
username: this.customer.email,
password: this.customer.password,
}
this.axios.post('/oauth/token', data)
.then(res => {
if (res.status == 200) { //如果成功了,就把a(bǔ)ccess_token存入localStorage
localStorage.token_type = res.data.token_type
localStorage.access_token = res.data.access_token
this.$router.push({name:'Index'})
}
})
}
}
}
/script>
客戶端查看 localStorage
,如圖:
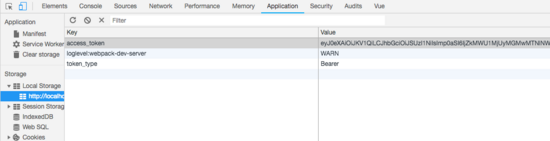
3、在 http.js
文件中設(shè)置攔截器,用于判斷用戶是否登錄,若沒有登錄跳轉(zhuǎn)到登錄頁面。代碼如下:
//#創(chuàng)建http.js文件
import axios from 'axios'
import router from '@/router'
// axios 配置
axios.defaults.timeout = 5000;
axios.defaults.baseURL = 'http://wechat.test/';
// http request 攔截器
axios.interceptors.request.use(
config => { //將所有的axios的header里加上token_type和access_token
config.headers.Authorization = `${localStorage.token_type} ${localStorage.access_token}`;
return config;
},
err => {
return Promise.reject(err);
});
// http response 攔截器
axios.interceptors.response.use(
response => {
return response;
},
error => {
// 401 清除token信息并跳轉(zhuǎn)到登錄頁面
if (error.response.status == 401) {
alert('您還沒有登錄,請先登錄')
router.replace({ //如果失敗,跳轉(zhuǎn)到登錄頁面
name: 'Login'
})
}
return Promise.reject(error.response.data)
});
export default axios;
重新訪問項(xiàng)目,在商品詳情頁面點(diǎn)擊加入購物車,你會(huì)發(fā)覺奇跡已經(jīng)出現(xiàn),當(dāng)你沒有登錄時(shí),提示跳轉(zhuǎn)到登錄頁面。輸入賬號密碼,登錄成功,此時(shí)就能拿到用戶id。接下來,繼續(xù)測試。
4、去 Cart
控制器中,找到購物車首頁方法,獲取用戶的id,獲取方式如下:
$customer_id = auth('api')->user()->id;
return $customer_id;
5、在 postman
中輸入購物車首頁接口地址,并傳入所需參數(shù),參數(shù)參考地址: http://laravelacademy.org/post/8909.html
,如圖:
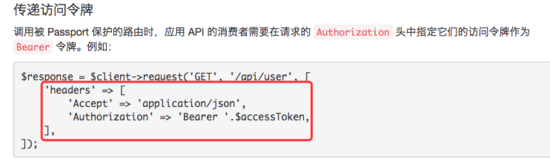
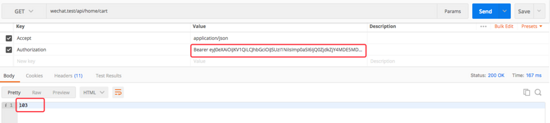
拿到用戶id后,把后端之前定義的customer_id全部改為通過接口方法獲取。至此, Passport
接口認(rèn)證的全部操作已完成。
總結(jié):接口認(rèn)證邏輯思想
1、安裝passport后,生成client_id和 client_secret
2、使用username、password、client_id、client_secret、grant_type參數(shù),調(diào)用/oauth/token接口,拿到access_token
3、需要認(rèn)證的接口,加上中間件。這時(shí)候直接訪問接口地址,會(huì)提示沒有認(rèn)證的。帶上access_token后,才能拿到接口的數(shù)據(jù)。
以上就是本文的全部內(nèi)容,希望對大家的學(xué)習(xí)有所幫助,也希望大家多多支持腳本之家。
您可能感興趣的文章:- laravel框架 api自定義全局異常處理方法
- 基于laravel制作APP接口(API)
- 基于Laravel Auth自定義接口API用戶認(rèn)證的實(shí)現(xiàn)方法
- 詳解laravel安裝使用Passport(Api認(rèn)證)
- laravel dingo API返回自定義錯(cuò)誤信息的實(shí)例
- 在 Laravel 中動(dòng)態(tài)隱藏 API 字段的方法
- Laravel如何實(shí)現(xiàn)適合Api的異常處理響應(yīng)格式