眾所周知canvas是位圖,在位圖里我們可以在里面畫各種東西,可以是圖片,可以是線條等等。那我們想給canvas里的某一張圖片添加一個(gè)點(diǎn)擊事件該怎么做到。而js只能監(jiān)聽到canvas的事件,很明顯這個(gè)圖片是不存在與dom里面的圖片只是畫在了canvas里而已。下面我就來簡(jiǎn)單的實(shí)現(xiàn)一個(gè)canvas內(nèi)部各個(gè)圖片的事件綁定。
我先來講下實(shí)現(xiàn)原理:其實(shí)就是canvas綁定相關(guān)事件,在通過記錄圖片所在canvas的坐標(biāo),判斷事件作用于哪個(gè)圖片中。這樣講是不是感覺跟事件代理有點(diǎn)相似咧。不過實(shí)現(xiàn)起來還是有稍許復(fù)雜的。
ps:下面的代碼我是用ts寫的,大家當(dāng)es6看就好了,稍有不同的可以查看
typescript的文檔 (typescript真的很好用,建議大家多多了解)。
1、建立圖片和canvas之間的聯(lián)系(這里我用色塊來代替圖片)
這里要色塊和canvas建立一定的聯(lián)系,而不是單純的渲染。還要記錄色塊所在坐標(biāo)、寬高。我們先一步一步來實(shí)現(xiàn)
首先寫基本的html頁(yè)面創(chuàng)建一個(gè)canvas:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>canvas事件</title>
<style>
html, body {
height: 100%;
background: #eee;
}
canvas {
background: #fff;
display: block;
margin: 0 auto;
}
</style>
</head>
<body>
<canvas width="500" height="500" id="canvas"></canvas>
</body>
下一步,我們要定一個(gè)Canvas的類,這個(gè)類應(yīng)該要有些什么功能呢?
- 要有對(duì)應(yīng)的canvas。
- 裝色塊數(shù)據(jù)的容器。
- 有添加色塊的方法。
- 渲染色塊的方法。
- 渲染所有色塊的方法。
因?yàn)樯珘K也有自己的一些參數(shù),為了方便拓展,我們也為色塊定一個(gè)類,這類需要的功能有:
寬、高、顏色、坐標(biāo)(x,y),還有Canvas實(shí)例;初步就定這幾個(gè)吧
ok開始寫
// Canvas類
class Canvas {
blockList: Block[]
ctx: any
canvas: any
createBlock (option) {
option.Canvas = this
this.blockList.push(new Block(option))
this.painting()
}
rendering (block) { // 渲染色塊函數(shù)
this.ctx.fillStyle = block.color
this.ctx.fillRect(block.x, block.y, block.w, block.h)
}
painting () { // 將容器里的色塊全部渲染到canvas
// 清空畫布(渲染之前應(yīng)該將老的清空)
this.ctx.fillStyle = '#fff'
this.ctx.fillRect(0, 0, this.canvas.width, this.canvas.height)
this.blockList.forEach(ele => {
this.rendering(ele)
})
}
constructor (ele) { // 初始化函數(shù)(輸入的是canvas)
// 設(shè)置canvas
this.canvas = ele
this.ctx = this.canvas.getContext('2d')
// 色塊容器
this.blockList = []
}
}
class Block {
w: number
h: number
x: number
y: number
color: string
Canvas: Canvas
hierarchy: number
constructor ({ w, h, x, y, color, Canvas }) { // 初始化設(shè)置色塊相關(guān)屬性
this.w = w
this.h = h
this.x = x
this.y = y
this.color = color
this.Canvas = Canvas
}
}
下面運(yùn)行一波試試
// 創(chuàng)建Canvas實(shí)例,并添加藍(lán)色個(gè)寬高100px,位置(100,100)、(300,100)紅色和藍(lán)色的色塊
var canvas = new Canvas(document.getElementById('canvas'))
canvas.createBlock({ // 紅色
x: 100,
y: 100,
w: 100,
h: 100,
color: '#f00'
})
canvas.createBlock({ // 藍(lán)色
x: 100,
y: 100,
w: 300,
h: 100,
color: '#00f'
})
運(yùn)行結(jié)果如下:
2、給色塊添加點(diǎn)擊事件
這里并不能直接給色塊添加點(diǎn)擊事件的,所以要通過坐標(biāo)的方式判斷目前點(diǎn)擊的是哪個(gè)色塊。
- 先給canvas添加點(diǎn)擊事件。
- 判斷色塊區(qū)域。
- 執(zhí)行相應(yīng)事件。
class Block {
// ...省略部分代碼
checkBoundary (x, y) { // 判斷邊界方法
return x > this.x && x < (this.x + this.w) && y > this.y && y < (this.y + this.h)
}
mousedownEvent () { // 點(diǎn)擊事件
console.log(`點(diǎn)擊了顏色為${this.color}的色塊`)
}
}
class Canvas {
// ...省略部分代碼
constructor (ele) {
this.canvas = ele
this.ctx = this.canvas.getContext('2d')
this.blockList = []
// 事件綁定(這里有一個(gè)要注意的,我這里用了bind方法,是為了將“mousedownEvent”方法內(nèi)的this指向切換到Canvas)
this.canvas.addEventListener('click', this.mousedownEvent.bind(this)) // 點(diǎn)擊事件
}
mousedownEvent () { // 點(diǎn)擊事件
const x = e.offsetX
const y = e.offsetY
// 這里將點(diǎn)擊的坐標(biāo)傳給所有色塊,根據(jù)邊界判斷方法判斷是否在點(diǎn)擊在內(nèi)部。是的話執(zhí)行色塊的事件方法。
this.blockList.forEach(ele => {
if (ele.checkBoundary(x, y)) ele.mousedownEvent(e)
})
}
}
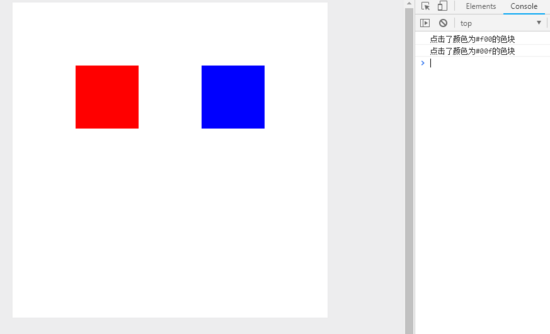
到這里為止已經(jīng)實(shí)現(xiàn)了對(duì)不同canvas內(nèi)不同色塊綁定對(duì)應(yīng)的點(diǎn)擊事件。不過這個(gè)點(diǎn)擊事件是不完美的,因?yàn)槟壳盀橹刮覀冞€沒有引入層級(jí)的概念,就是說兩個(gè)色塊重疊部分點(diǎn)擊的話,全部都會(huì)觸發(fā)。所以我們還要給色塊加入層級(jí)的屬性。實(shí)現(xiàn)一個(gè)點(diǎn)擊某一個(gè)色塊改色塊的層級(jí)就會(huì)提升到最高。
class Block {
// ...省略部分代碼
constructor ({ w, h, x, y, color, Canvas, hierarchy }) { // 初始化設(shè)置色塊相關(guān)屬性
this.w = w
this.h = h
this.x = x
this.y = y
this.color = color
this.Canvas = Canvas
this.hierarchy = 0
}
}
class Canvas {
// ...省略部分代碼
constructor (ele) {
this.canvas = ele
this.ctx = this.canvas.getContext('2d')
this.blockList = []
// 事件綁定(這里有一個(gè)要注意的,我這里用了bind方法,是為了將“mousedownEvent”方法內(nèi)的this指向切換到Canvas)
this.canvas.addEventListener('click', this.mousedownEvent.bind(this)) // 點(diǎn)擊事件
this.nowBlock = null // 當(dāng)前選中的色塊
}
createBlock (option) { // 創(chuàng)建色塊函數(shù)(這里的Block是色塊的類)
option.Canvas = this
// 創(chuàng)建最新的色塊的層級(jí)應(yīng)該是最高的
option.hierarchy = this.blockList.length
this.blockList.push(new Block(option))
this.rendering()
}
mousedownEvent (e) { // 點(diǎn)擊事件
const x = e.offsetX
const y = e.offsetY
// 獲取點(diǎn)中里層級(jí)最高的色塊
this.nowBlock = (this.blockList.filter(ele => ele.checkBoundary(x, y))).pop()
// 如果沒有捕獲的色塊直接退出
if (!this.nowBlock) return
// 將點(diǎn)擊到的色塊層級(jí)提高到最高
this.nowBlock.hierarchy = this.blockList.length
// 重新排序(從小到大)
this.blockList.sort((a, b) => a.hierarchy - b.hierarchy)
// 在重新從0開始分配層級(jí)
this.blockList.forEach((ele, idx) => ele.hierarchy = idx)
// 重新倒序排序后再重新渲染。
this.painting()
this.nowBlock.mousedownEvent(e) // 只觸發(fā)選中的色塊的事件
}
}
// 這里我們還得加入第三塊色塊與紅色色塊重疊的色塊
canvas.createBlock({
x: 150,
y: 150,
w: 100,
h: 100,
color: '#0f0'
})
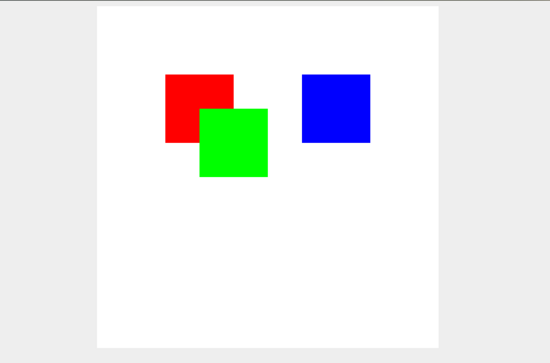
Canvas中“mousedownEvent”方法內(nèi)的代碼是有點(diǎn)復(fù)雜的,主要是有點(diǎn)繞。
- 首先是this.nowBlock = (this.blockList.filter(ele => ele.checkBoundary(x, y))).pop()這段代碼是怎么獲取到點(diǎn)擊到的色塊中層級(jí)最高的色塊。這里因?yàn)槲覀兠看翁砑由珘K都是設(shè)置了最高層級(jí)的,所以“blockList”內(nèi)的色塊都是按層級(jí)從小到大排序的。所以我們?nèi)∽詈笠粋€(gè)就可以了。
- 第二步就是將拿到的色塊的層級(jí)提升到最高。
- 第三步就是從小到大重新排列色塊。
- 因?yàn)榈诙降臅r(shí)候我們修改了選中色塊的層級(jí),導(dǎo)致所有色塊的層級(jí)不是連續(xù)的,為了避免層級(jí)不可控,我們還得重新定義層級(jí)。
- 重新渲染色塊到canvas中,因?yàn)?ldquo;blockList”內(nèi)的色塊是排好序的,所以按順序渲染即可。
運(yùn)行后的效果就是下面這樣了:
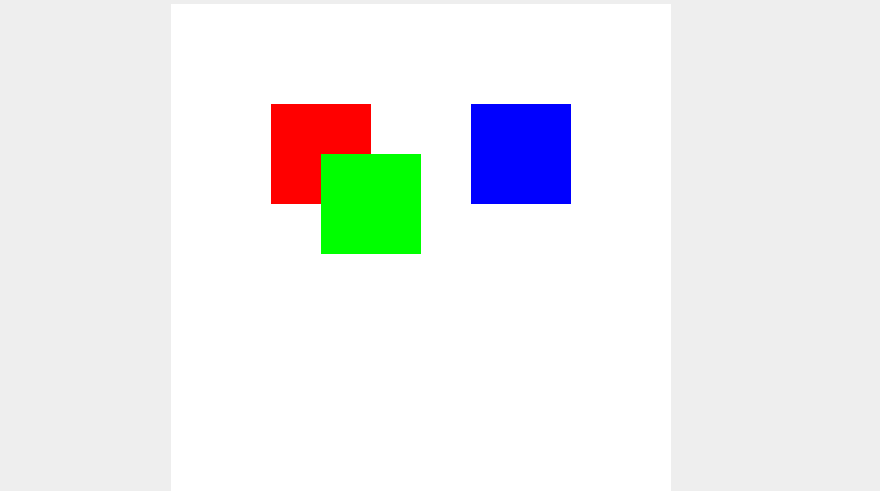
3、實(shí)現(xiàn)對(duì)不同色塊進(jìn)行拖拽
在上面我們已經(jīng)實(shí)現(xiàn)了獲取不同的色塊,并修改它的層級(jí)。下面我們要實(shí)現(xiàn)色塊的拖拽,主要就是獲取鼠標(biāo)移動(dòng)過程中和一開始點(diǎn)擊下去時(shí)位置坐標(biāo)的變化。這個(gè)原理和普通的dom拖拽實(shí)現(xiàn)原理一樣。
獲取點(diǎn)擊色塊的點(diǎn),距離色塊左邊和上邊的距離(disX, disY)。
鼠標(biāo)移動(dòng)時(shí),用鼠標(biāo)當(dāng)前距離canvas左邊和上邊的距離減去(disX, disY)這里就是色塊的x,y坐標(biāo)了。
class Block {
// ...省略部分代碼
mousedownEvent (e: MouseEvent) {
/* 這里 disX和disY的計(jì)算方式: e.offsetX獲取到的是鼠標(biāo)點(diǎn)擊距離canvas左邊的距離,this.x是色塊距離canvas左邊的距離。e.offsetX-this.x就是色塊左邊的距離。這應(yīng)該很好理解了 */
const disX = e.offsetX - this.x // 點(diǎn)擊時(shí)距離色塊左邊的距離
const disY = e.offsetY - this.y // 點(diǎn)擊時(shí)距離色塊上邊的距離
// 綁定鼠標(biāo)滑動(dòng)事件;這里mouseEvent.offsetX同樣是鼠標(biāo)距離canvas左側(cè)的距離,mouseEvent.offsetX - disX就是色塊的x坐標(biāo)了。同理y也是這樣算的。最后在重新渲染就好了。
document.onmousemove = (mouseEvent) => {
this.x = mouseEvent.offsetX - disX
this.y = mouseEvent.offsetY - disY
this.Canvas.painting()
}
// 鼠標(biāo)松開則清空所有事件
document.onmouseup = () => {
document.onmousemove = document.onmousedown = null
}
// console.log(`點(diǎn)擊了顏色為${this.color}的色塊22`)
}
}
效果如下:
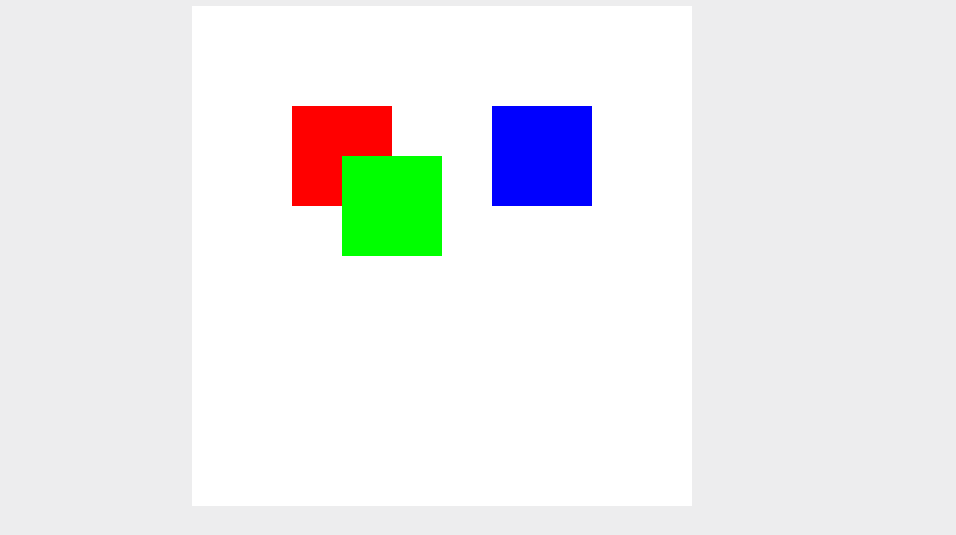
下面貼上完整的代碼(html和調(diào)用的方法就不放了)這個(gè)例子只是簡(jiǎn)單實(shí)現(xiàn)給canvas內(nèi)的內(nèi)容綁定事件,大家可以實(shí)現(xiàn)復(fù)雜一點(diǎn)的,例如把色塊換成圖片,除了拖拽還以給圖片縮放,旋轉(zhuǎn),刪除等等。
class Canvas {
blockList: Block[]
ctx: any
canvas: any
nowBlock: Block
createBlock (option) {
option.hierarchy = this.blockList.length
option.Canvas = this
this.blockList.push(new Block(option))
this.painting()
}
rendering (block) {
this.ctx.fillStyle = block.color
this.ctx.fillRect(block.x, block.y, block.w, block.h)
}
painting () {
// 清空畫布
this.ctx.fillStyle = '#fff'
this.ctx.fillRect(0, 0, this.canvas.width, this.canvas.height)
this.blockList.forEach(ele => {
this.rendering(ele)
})
}
mousedownEvent (e: MouseEvent) { // 點(diǎn)擊事件
const x = e.offsetX
const y = e.offsetY
// 獲取點(diǎn)中里層級(jí)最高的色塊
this.nowBlock = (this.blockList.filter(ele => ele.checkBoundary(x, y))).pop()
// 如果沒有捕獲的色塊直接退出
if (!this.nowBlock) return
// 將點(diǎn)擊到的色塊層級(jí)提高到最高
this.nowBlock.hierarchy = this.blockList.length
// 重新排序(從小到大)
this.blockList.sort((a, b) => a.hierarchy - b.hierarchy)
// 在重新從0開始分配層級(jí)
this.blockList.forEach((ele, idx) => ele.hierarchy = idx)
// 重新倒序排序后再重新渲染。
this.painting()
this.nowBlock.mousedownEvent(e)
// this.blockList.forEach(ele => {
// if (ele.checkBoundary(x, y)) ele.clickEvent(e)
// })
}
constructor (ele) {
this.canvas = ele
this.ctx = this.canvas.getContext('2d')
this.blockList = []
// 事件綁定
this.canvas.addEventListener('mousedown', this.mousedownEvent.bind(this))
}
}
class Block {
w: number
h: number
x: number
y: number
color: string
Canvas: Canvas
hierarchy: number
constructor ({ w, h, x, y, color, Canvas, hierarchy }) {
this.w = w
this.h = h
this.x = x
this.y = y
this.color = color
this.Canvas = Canvas
this.hierarchy = hierarchy
}
checkBoundary (x, y) {
return x > this.x && x < (this.x + this.w) && y > this.y && y < (this.y + this.h)
}
mousedownEvent (e: MouseEvent) {
const disX = e.offsetX - this.x
const disY = e.offsetY - this.y
document.onmousemove = (mouseEvent) => {
this.x = mouseEvent.offsetX - disX
this.y = mouseEvent.offsetY - disY
this.Canvas.painting()
}
document.onmouseup = () => {
document.onmousemove = document.onmousedown = null
}
// console.log(`點(diǎn)擊了顏色為${this.color}的色塊22`)
}
}
以上就是本文的全部?jī)?nèi)容,希望對(duì)大家的學(xué)習(xí)有所幫助,也希望大家多多支持腳本之家。